HelloWorld¶
The basic HelloWorld example describes the steps necessary for setting up DCPS entities (see also HelloWorld tutorial).
Note
The HelloWorld example is referenced throughout the Getting Started Guide.
Design¶
The HelloWorld example consists of two units:
HelloworldPublisher: implements the publisher’s main
HelloworldSubscriber: implements the subscriber’s main
Scenario¶
The publisher sends a single HelloWorld sample. The sample contains two fields:
a
userID
field (long type)a
message
field (string type)
When it receives the sample, the subscriber displays the userID
and the message
field.
Running the example¶
To avoid mixing the output, run the subscriber and publisher in separate terminals.
Open two terminals inside the directory with the HelloWorld files.
In the first terminal, start the subscriber by running
HelloWorldSubscriber
../HelloworldSubscriber
./HelloworldSubscriber
HelloworldSubscriber.exe
In the second terminal, start the publisher by running
HelloWorldPublisher
../HelloworldPublisher
./HelloworldPublisher
HelloworldPublisher.exe
HelloworldPublisher
appears as follows:
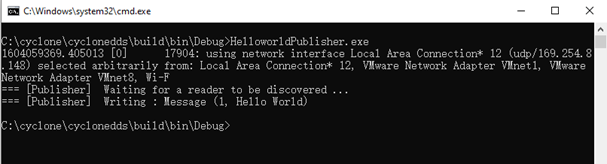
HelloworldSubscriber
appears as follows:
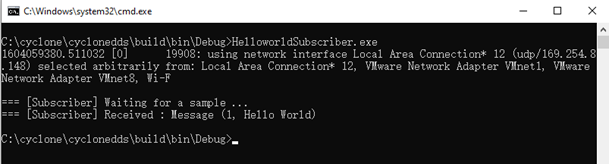
For the source code, refer to the HelloWorld source code.
Source code¶
1module HelloWorldData
2{
3 struct Msg
4 {
5 @key
6 long userID;
7 string message;
8 };
9};
1#include "dds/dds.h"
2#include "HelloWorldData.h"
3#include <stdio.h>
4#include <stdlib.h>
5
6int main (int argc, char ** argv)
7{
8 dds_entity_t participant;
9 dds_entity_t topic;
10 dds_entity_t writer;
11 dds_return_t rc;
12 HelloWorldData_Msg msg;
13 uint32_t status = 0;
14 (void)argc;
15 (void)argv;
16
17 /* Create a Participant. */
18 participant = dds_create_participant (DDS_DOMAIN_DEFAULT, NULL, NULL);
19 if (participant < 0)
20 DDS_FATAL("dds_create_participant: %s\n", dds_strretcode(-participant));
21
22 /* Create a Topic. */
23 topic = dds_create_topic (
24 participant, &HelloWorldData_Msg_desc, "HelloWorldData_Msg", NULL, NULL);
25 if (topic < 0)
26 DDS_FATAL("dds_create_topic: %s\n", dds_strretcode(-topic));
27
28 /* Create a Writer. */
29 writer = dds_create_writer (participant, topic, NULL, NULL);
30 if (writer < 0)
31 DDS_FATAL("dds_create_writer: %s\n", dds_strretcode(-writer));
32
33 printf("=== [Publisher] Waiting for a reader to be discovered ...\n");
34 fflush (stdout);
35
36 rc = dds_set_status_mask(writer, DDS_PUBLICATION_MATCHED_STATUS);
37 if (rc != DDS_RETCODE_OK)
38 DDS_FATAL("dds_set_status_mask: %s\n", dds_strretcode(-rc));
39
40 while(!(status & DDS_PUBLICATION_MATCHED_STATUS))
41 {
42 rc = dds_get_status_changes (writer, &status);
43 if (rc != DDS_RETCODE_OK)
44 DDS_FATAL("dds_get_status_changes: %s\n", dds_strretcode(-rc));
45
46 /* Polling sleep. */
47 dds_sleepfor (DDS_MSECS (20));
48 }
49
50 /* Create a message to write. */
51 msg.userID = 1;
52 msg.message = "Hello World";
53
54 printf ("=== [Publisher] Writing : ");
55 printf ("Message (%"PRId32", %s)\n", msg.userID, msg.message);
56 fflush (stdout);
57
58 rc = dds_write (writer, &msg);
59 if (rc != DDS_RETCODE_OK)
60 DDS_FATAL("dds_write: %s\n", dds_strretcode(-rc));
61
62 /* Deleting the participant will delete all its children recursively as well. */
63 rc = dds_delete (participant);
64 if (rc != DDS_RETCODE_OK)
65 DDS_FATAL("dds_delete: %s\n", dds_strretcode(-rc));
66
67 return EXIT_SUCCESS;
68}
1#include "dds/dds.h"
2#include "HelloWorldData.h"
3#include <stdio.h>
4#include <string.h>
5#include <stdlib.h>
6
7/* An array of one message (aka sample in dds terms) will be used. */
8#define MAX_SAMPLES 1
9
10int main (int argc, char ** argv)
11{
12 dds_entity_t participant;
13 dds_entity_t topic;
14 dds_entity_t reader;
15 HelloWorldData_Msg *msg;
16 void *samples[MAX_SAMPLES];
17 dds_sample_info_t infos[MAX_SAMPLES];
18 dds_return_t rc;
19 dds_qos_t *qos;
20 (void)argc;
21 (void)argv;
22
23 /* Create a Participant. */
24 participant = dds_create_participant (DDS_DOMAIN_DEFAULT, NULL, NULL);
25 if (participant < 0)
26 DDS_FATAL("dds_create_participant: %s\n", dds_strretcode(-participant));
27
28 /* Create a Topic. */
29 topic = dds_create_topic (
30 participant, &HelloWorldData_Msg_desc, "HelloWorldData_Msg", NULL, NULL);
31 if (topic < 0)
32 DDS_FATAL("dds_create_topic: %s\n", dds_strretcode(-topic));
33
34 /* Create a reliable Reader. */
35 qos = dds_create_qos ();
36 dds_qset_reliability (qos, DDS_RELIABILITY_RELIABLE, DDS_SECS (10));
37 reader = dds_create_reader (participant, topic, qos, NULL);
38 if (reader < 0)
39 DDS_FATAL("dds_create_reader: %s\n", dds_strretcode(-reader));
40 dds_delete_qos(qos);
41
42 printf ("\n=== [Subscriber] Waiting for a sample ...\n");
43 fflush (stdout);
44
45 /* Initialize sample buffer, by pointing the void pointer within
46 * the buffer array to a valid sample memory location. */
47 samples[0] = HelloWorldData_Msg__alloc ();
48
49 /* Poll until data has been read. */
50 while (true)
51 {
52 /* Do the actual read.
53 * The return value contains the number of read samples. */
54 rc = dds_read (reader, samples, infos, MAX_SAMPLES, MAX_SAMPLES);
55 if (rc < 0)
56 DDS_FATAL("dds_read: %s\n", dds_strretcode(-rc));
57
58 /* Check if we read some data and it is valid. */
59 if ((rc > 0) && (infos[0].valid_data))
60 {
61 /* Print Message. */
62 msg = (HelloWorldData_Msg*) samples[0];
63 printf ("=== [Subscriber] Received : ");
64 printf ("Message (%"PRId32", %s)\n", msg->userID, msg->message);
65 fflush (stdout);
66 break;
67 }
68 else
69 {
70 /* Polling sleep. */
71 dds_sleepfor (DDS_MSECS (20));
72 }
73 }
74
75 /* Free the data location. */
76 HelloWorldData_Msg_free (samples[0], DDS_FREE_ALL);
77
78 /* Deleting the participant will delete all its children recursively as well. */
79 rc = dds_delete (participant);
80 if (rc != DDS_RETCODE_OK)
81 DDS_FATAL("dds_delete: %s\n", dds_strretcode(-rc));
82
83 return EXIT_SUCCESS;
84}